In the ever-evolving landscape of web applications and APIs, security is paramount. OAuth 2.0 has emerged as a standard protocol for securing and authorizing access to resources across platforms. At its core, OAuth 2.0 facilitates the delegation of access without sharing user credentials. In this article, we’ll delve into the world of OAuth 2.0 token generation, accompanied by practical code snippets to help you implement this process effectively.
Understanding OAuth 2.0
OAuth 2.0 defines several grant types, each serving a specific use case. The most common ones include:
- Authorization Code Grant: Used by web applications that can securely store a client secret.
- Implicit Grant: Designed for mobile apps and web applications unable to store a client secret.
- Client Credentials Grant: Suitable for machine-to-machine communication where no user is involved.
- Resource Owner Password Credentials Grant: Appropriate when the application fully trusts the user with their credentials.
For this article, we’ll focus on the Authorization Code Grant, often used in scenarios involving web applications.
Token Generation: Step by Step
- User Authorization Request: The user requests access to a resource, and the application redirects them to the authorization server.
- User Authorization: The user logs in and grants permissions to the application.
- Authorization Code Request: The application requests an authorization code from the authorization server.
- Authorization Code Response: The authorization server responds with an authorization code.
- Token Request: The application exchanges the authorization code for an access token.
- Token Response: The authorization server returns an access token and optionally, a refresh token.
Sample Code Snippets
Let’s walk through the process with some code snippets using a hypothetical web application written in Python using the Flask framework.
1. User Authorization Request:
python
from flask import Flask, redirect
app = Flask(__name__)
@app.route(‘/login’)
def login():
# Redirect user to authorization server for login and consent
authorization_url = ‘https://auth-server.com/authorize‘
return redirect(authorization_url)
if __name__ == ‘__main__’:
app.run()
2. Authorization Code Response:
python
from flask import Flask, request
app = Flask(__name__)
@app.route(‘/callback’)
def callback():
authorization_code = request.args.get(‘code’)
# Send authorization code to the server for token exchange
# …
if __name__ == ‘__main__’:
app.run()
python
import requests
authorization_code = ‘your_authorization_code’
token_url = ‘https://auth-server.com/token‘
client_id = ‘your_client_id’
client_secret = ‘your_client_secret’
data = {
‘grant_type’: ‘authorization_code’,
‘code’: authorization_code,
‘client_id’: client_id,
‘client_secret’: client_secret,
‘redirect_uri’: ‘http://yourapp.com/callback‘
}
response = requests.post(token_url, data=data)
access_token = response.json()[‘access_token’]
Now you have the access token that your application can use to access the user’s resources.
In conclusion, OAuth 2.0 token generation is a crucial process for securing your web applications and APIs. By following the steps and utilizing the code snippets provided, you can seamlessly integrate OAuth 2.0 into your projects, enhancing both security and user experience. Always ensure that you are following best practices and guidelines to ensure the safety of your users’ data and resources.
In the ever-evolving landscape of web applications and APIs, security is paramount. OAuth 2.0 has emerged as a standard protocol for securing and authorizing access to resources across platforms. At its core, OAuth 2.0 facilitates the delegation of access without sharing user credentials. In this article, we’ll delve into the world of OAuth 2.0 token generation, accompanied by practical code snippets to help you implement this process effectively.
Understanding OAuth 2.0
OAuth 2.0 defines several grant types, each serving a specific use case. The most common ones include:
- Authorization Code Grant: Used by web applications that can securely store a client secret.
- Implicit Grant: Designed for mobile apps and web applications unable to store a client secret.
- Client Credentials Grant: Suitable for machine-to-machine communication where no user is involved.
- Resource Owner Password Credentials Grant: Appropriate when the application fully trusts the user with their credentials.
For this article, we’ll focus on the Authorization Code Grant, often used in scenarios involving web applications.
Token Generation: Step by Step
- User Authorization Request: The user requests access to a resource, and the application redirects them to the authorization server.
- User Authorization: The user logs in and grants permissions to the application.
- Authorization Code Request: The application requests an authorization code from the authorization server.
- Authorization Code Response: The authorization server responds with an authorization code.
- Token Request: The application exchanges the authorization code for an access token.
- Token Response: The authorization server returns an access token and optionally, a refresh token.
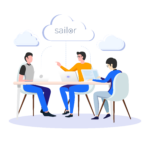
- Google OAuth 2.0 documentation: https://developers.google.com/identity/protocols/oauth2
- Microsoft OAuth 2.0 authorization code flow: https://learn.microsoft.com/en-us/entra/identity-platform/v2-oauth2-auth-code-flow
- Auth0 OAuth 2.0 token generation guide: https://auth0.com/docs/authenticate/protocols/oauth